Inheritance is a process in which a class acquiers the properties as well as behaviour of another class. In this, the class which acquires the properties is called Subclass and the class from which subclass acquires the properties is called Superclass.
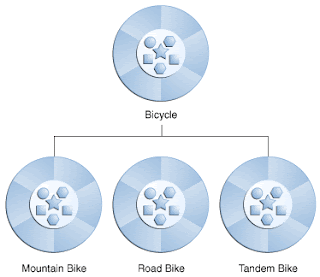
Inheritance can be of 5 types :
1. Single Inheritance
2. Multiple Inheritance
3. Multi-level Inheritance
4. Hierarchial Inheritance
5. Network Inheritance
JAVA doesnot supports Multiple Inheritance. Instead of Multiple Inheritance, it uses another concept termed as Interfacing.
To inherit the properties we use extends key word. Supposewe have class A and class B.
public class A
{
.........
........
.......
}
public class B extends A
{.....
........
......
}
Here class B inherits the properties of A.
To inherit the properties we use extends key word. Supposewe have class A and class B.
public class A
{
.........
........
.......
}
public class B extends A
{.....
........
......
}
Here class B inherits the properties of A.
example:-
// A simple example of inheritance.
// Create a superclass.
class A {
int i, j;
void showij() {
System.out.println("i and j: " + i + " " + j);
}
}
// Create class B extending class A.
class B extends A {
int k;
void showk() {
System.out.println("k: " + k);
}
void sum() {
System.out.println("i+j+k: " + (i+j+k));
}
}
class SimpleInheritance {
public static void main(String args[])
{
A superOb = new A();
B subOb = new B();
superOb.i = 10;
superOb.j = 20;
System.out.println("Contents of superOb: ");
superOb.showij();
System.out.println();
subOb.i = 7;
subOb.j = 8;
subOb.k = 9;
System.out.println("Contents of subOb: ");
subOb.showij();
subOb.showk();
System.out.println();
System.out.println("Sum of i, j and k in subOb:");
subOb.sum();
}
}
The output from this program is :
Contents of superOb:
i and j: 10 20
Contents of subOb:
i and j: 7 8
k: 9
Sum of i, j and k in subOb:
i+j+k: 24
0 comments:
Post a Comment